티스토리 뷰
728x90
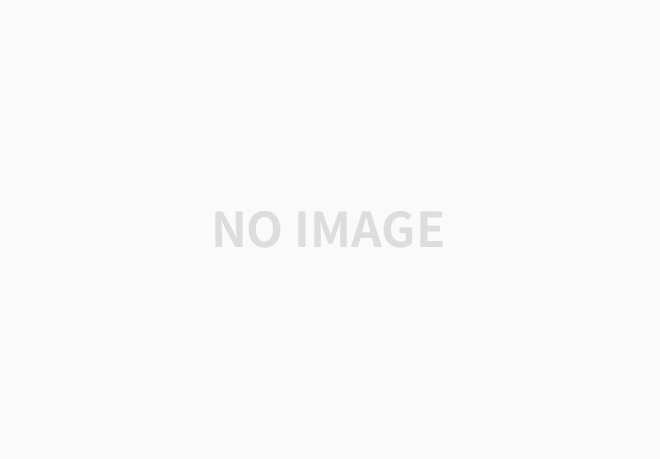
메모리 구조
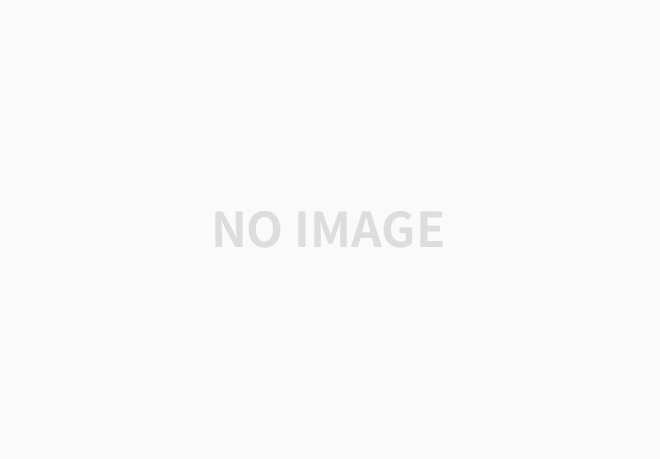
변수
매개변수
- 기본형이면, 값이 복사된다. (read only)
- 참조형이면, 인스턴스 주소가 복사된다. (read & write)
스태틱(클래식) 변수와 인스턴스 변수
public class Card {
// 스태틱(클래스) 변수 : 모든 인스턴스에 공통으로 들어가는 성질
static int height = 88;
static int width = 62;
// 인스턴스 변수 : 인스턴스별로 다른 성질
// 클래스 메서드가 사용할 수 없다.
int number;
String shape;
Card(){
this.number = 1;
this.shape = "Spade";
}
Card(int number, String shape){
this.number = number;
this.shape = shape;
}
@Override
public String toString() {
return "Card [number=" + this.number + ", shape=" + this.shape + "]";
}
}
Class
Class객체
클래스의 모든 정보를 담고 있으며 클래스당 1개만 존재
클래스로더
실행 시에 필요한 클래스를 동적으로 메모리에 로드
초기화 블록
클래스 초기화 블록
해당 클래스가 메모리에 처음 로딩될 때 한 번만 수행된다. → static { ... }
인스턴스 초기화 블록
인스턴스가 생성될 때마다 수행된다. → { ... }
class InitTest{
// cv : class variable 클래스 변수
// iv : instance variable 인스턴스 변수
// [순서]
// 1. 기본값
// 2. 명시적 초기화
static int cv = 1;
int iv = 1;
// 3. 클래스 초기화 블럭
static {
cv = 2;
}
// 3. 인스턴스 초기화 블럭
{
iv = 2;
}
// 4. 생성자(인스턴스)
InitTest() {
iv = 3;
}
}
반복문
무한 반복문
for(;;) {
// code here
}
while(true) {
// code here
}
do-while문
최소 한 번은 무조건 수행된다는 특징이 있다.
조건식 뒤에 ';'에 주의해야 한다.
do {
// code here
} while (조건식);
이름 붙은 반복문
하나 이상의 반복문도 벗어날 수 있다.
// i가 5가되면 이중반복문 Loop1를 벗어나게 된다.
int i = 0;
Loop1 : for(;;) {
for(;;) {
if (i == 5){
break Loop1;
}
i++;
}
}
그 외
- 조건식의 연산속도
- OR 연산의 경우, '참'일 확률이 높은 피연산자를 먼저 기술하는 것이 더 빠른 연산 결과를 얻을 수 있다.
- char 배열 → String
char[] charArr = new char[]{'H','e','l','l','o'};
String str = new String(charArr);
System.out.println(str); // Hello
- 배열의 선언과 생성
int[] nums1 = new int[] {1, 2, 3};
int[] nums2 = {1, 2, 3}; // new int[] 생략 가능
System.out.println(Arrays.toString(nums1)); // [1, 2, 3]
System.out.println(Arrays.toString(nums2)); // [1, 2, 3]
- 한 번 생성한 배열은 길이를 변경할 수 없으므로, 배열의 length는 상수이다.
- 배열을 복사하는데에는 for문보다 System.arraycopy()가 빠르다.
728x90
'KR > Java' 카테고리의 다른 글
놓치기 쉬운 자바(JAVA) : (3) java.lang 패키지 (0) | 2021.06.11 |
---|---|
놓치기 쉬운 자바(JAVA) : (2) 객체지향 (0) | 2021.04.22 |
[Lombok] 롬복 설치 및 STS(eclipse) 연동하기 (1) | 2020.02.28 |
[Spring] 스프링 프레임워크(Spring Framework)를 사용하는 이유 (0) | 2019.10.02 |
[SpringBoot] Undertow 적용 및 배포 (1) | 2019.08.12 |